Integration FAQs
SDK Initialization
Q. How I can see the Netcore SDK's log?
If you are using SDK version v2.5.2 and higher, the logs are disabled by default. To enable the logs you can use setDebugLevel() method of NetcoreSDK.
public class MyApplication extends Application {
@Override
public void onCreate() {
super.onCreate();
Smartech smartech = Smartech.getInstance(new WeakReference<>(this));
smartech.initializeSdk(this);
smartech.setDebugLevel(NCLogger.Level.LOG_LEVEL_VERBOSE);
}
}
class MyApplication : Application() {
override fun onCreate() {
super.onCreate()
val smartech = getInstance(WeakReference(this))
smartech.initializeSdk(this)
smartech.setDebugLevel(NCLogger.Level.LOG_LEVEL_VERBOSE)
}
}
If you are using an older version of NetcoreSDK, the logs are visible in Android Logcat by default. You can find it by filtering the Logcat with "netcore".
Q. Why register event is not working?
Register event is responsible for initializing the Netcore SDK and passing the device's FCM token to the Smartech server. Failure of this event may cause in failure of Push Notifications and other SDK events. The following could be the reasons for the failure of the register event.
1. The register event is not called.
Make sure you are calling the register event of Netcore SDK in your application class.
public class MyApplication extends Application {
@Override
public void onCreate() {
super.onCreate();
Smartech smartech = Smartech.getInstance(new WeakReference<>(this));
smartech.initializeSdk(this);
}
}
class MyApplication : Application() {
override fun onCreate() {
super.onCreate()
val smartech = getInstance(WeakReference(this))
smartech.initializeSdk(this)
}
}
2. The application class is not registered in the AndroidManifest.
You need to register the application class in the AndroidManifest in order to invoke the onCreate() method of your application class.
<application
android:name=".MyApplication"
android:label="@string/app_name"
...>
3. The FCM token is null or empty.
If SDK receives a null or empty token for FCM, the register event will not work. To solve this issue check if you have called the setPushToken() method in the onNewToken() method of Firebase Messaging Service. Also if you are an existing user of FCM then you need to call the setPushToken() method in application class as well.
Refer to the document Send FCM token to Netcore SDK
Push Notifications
Q. Cannot see push icon?
The following could be the reasons.
1. Wrong syntax or No implementation.
Check if you have added the correct implementation for setting the small notification icon in the app level AndroidManifest.xml.
<!-- Syantax -->
<meta-data
android:name="SMT_SMALL_NOTIFICATION_ICON"
android:value="<small_notification_icon_name>"/>
<!-- Example -->
<meta-data
android:name="SMT_SMALL_NOTIFICATION_ICON"
android:value="ic_notif"/>
2. Icon not present in the drawable directory.
The drawable file must be present inside the res/drawable directory.
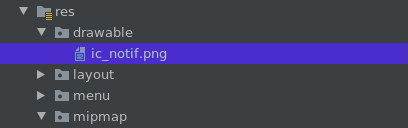
3. Icon not supported.
If the icon is been using is not as per Google’s guidelines for push icons, the icon will not display.
The icon size should match the following dimensions.
Type | Size (px) |
---|---|
Small Notification Icon (mdpi) | 24x24 |
Small Notification Icon (hdpi) | 36x36 |
Small Notification Icon (xhdpi) | 48x48 |
Small Notification Icon (xxhdpi) | 72x72 |
Small Notification Icon (xxxhdpi) | 96x96 |
Note: If your icon size matches the above dimensions but still the icon is not working then the icon must not be the transparent icon.
Q. Why Push notifications are not working?
The following could be the reasons.
1. handleNotification() not integrated or not accessible.
The handleNotification() method of SDK is responsible for handling the PNs received from Smartech. If the method is not implemented in the onMessageReceived() method of custom FCM service, the PNs will not work. If you have added some conditions before this method, confirm the handleNotification() method is accessible. The crash or exception in the FCM service may also cause the handleNotification() to be inaccessible.
2. FCM service not registered in the AndroidManifest.
Check if you have added your FCMService in the AndroidManifest of your app.
<service
android:name=".java.MyFirebaseMessagingService"
android:exported="false">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT" />
</intent-filter>
</service>
3. The register event is not working.
The register event sends the FCM token to the Smartech server. Smartech server use this token send push notifications to the device. If the register event fails, the Smartech server will not be able to sent push notifications.
To ensure the register event is working refer to the question "Why register event is not working?" in the SDK Initialization section of this document.
Q. Why Deeplinks are not working?
Users will be redirected to the home screen if the deep link is wrong. The deep link should be the combination of scheme and host specified in the data tag of intent-filters.
<activity
android:name=".MyCartActivity">
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data
android:host="my_cart"
android:scheme="https" />
</intent-filter>
</activity>
For the above snippet, the deep link would be “https://my_cart”.
Q. Campaign/Test push failed?
The following could be the reasons.
1. Token not found in database.
This error comes when Smartech is unable to find the token in its database. Sometimes due to the request queue on the Smartech server, tokens might not have been recorded to the Smartech database. In this case wait for 5-15 minutes to perform the next push. If you are still facing the issue, make sure the register event is happening on the device. To ensure the register event is working refer to the question "Why register event is not working?" in the SDK Initialization section of this document.
2. MismatchSenderId
This error comes when the google-services.json and the FCM server key saved at Smartech does not match. Make sure you are using a valid server key with respect to the google-services.json added in the Android app.
3. NotRegistered
When the targeted token no longer exists, i.e.the app is uninstalled, this error will be shown.
4. No reachable devices
This error will be popped when Smartech finds there are no reachable devices for the campaign. Try to refresh the reachable users in the audience section while creating the campaign.
Q. Why low delivery rates?
The following could be the reasons.
1. handleNotification() not integrated or not accessible.
Refer to the question "Why push notifications are not working?" in the Push Notifications section of this document.
2. FCM token is inaccessible to the SDK.
Make sure you are passing the valid token to NetcoreSDK. Also if you think before integration of NetcoreSDK, your previous app version already has FCM integrated into it, you need to get the existing FCM token and pass it to Netcore SDK using setPushToken() method in your application class.
Refer to "If you are already integrated with FCM" in the following document Send FCM token to Netcore SDK
In-Apps
Q. Why in-apps are not working?
1. Event name or parameter not matching.
Sometimes the event name or parameter name/value is different than that of in-app campaign. Make sure you are passing the correct details in custom event.
2. Context or Screen changed before In-App.
Suppose you are trying to trigger an event for which in-app is set. If another screen/activity opens before in-app, the in-app will not show. Example, Developer has set in-app for an event on SplashScreen activity, after triggering the event he/she is navigating the user to another activity. In this case, due to context/activity changing in-app will not open.
Q. Why in-apps deep links are not working?
Refer to the question "Why Deeplinks are not working?" in Push Notifications section.
Tracking User
Q. Cannot see user data on Smartech panel?
The following could be the reasons.
1. Invalid user identity.
If you pass null or blank identity in setIdentity() method of Netcore SDK, the SDK will consider it an anonymous user. In this case, all activities of the user are considered anonymous activities and cause no updates in the unified view of the user. Make sure you are passing valid value to setIdentity() method.
2. Wrong user identity.
Suppose the primary key set on the Smartech panel is EMAIL, and you are passing MOBILE in the setIdentity() method. In this case, the events/activities from that user would be considered as invalid and would be dropped by the Smartech server. You need to make sure that you are passing the same identity as the primary key set on the Smartech panel.
Q. Cannot see user profile data on Smartech panel.
The following could be the reasons.
1. Invalid user identity.
Refer to the question "Cannot see user data on Smartech panel?" in this section.
2. Wrong user identity.
Refer to the question "Cannot see user data on Smartech panel?" in this section.
3. The attribute is not created on the Smartech panel.
If the attribute that you are passing in the profile push data is not created on the panel, the profile push data will not reflect. You need to create the attributes on the panel for the same you are passing in profile push.
Tracking Event
Q. Event is not getting listed in Activities on Smartech panel.
This issue could happen if you pass invalid payload data for custom events. If the payload does not meet the below points then it is not a valid payload.
- The values inside the payload JSONObject must not be boolean.
- The key names inside the payload JSONObject must not contain a dot (“.”).
- The JSONObject and JSONArray in payload must not contain more than 2 level nesting if payload nesting is more than two-level, Smartech panel may discard the event.
Q. Even is not getting reflected in the unified view of user on Smartech panel.
The following could be the reasons.
1. Invalid user identity.
Refer to the question "Cannot see user data on Smartech panel?" in this section.
2. Wrong user identity.
Refer to the question "Cannot see user data on Smartech panel?" in this section.
Updated over 4 years ago