Nudges - Sending Nudges Events To Your Analytics System
Please follow the below steps if you wish to get nudge events shared back to your own analytics system.
Step 1 : Create a class that implements the HanselInternalEventsListener with the following method:
import io.hansel.ujmtracker.HanselInternalEventsListener;
HanselInternalEventsListener hanselInternalEventsListener = new HanselInternalEventsListener() {
@Override
public void onEvent(String eventName, HashMap dataFromHansel) {
if ("hansel_nudge_event".equals(eventName)){
// send `dataFromHansel` to your analytics provider
// e.g. CleverTap.push(clevertapAPI, "hansel_nudge_event", dataFromHansel);
}
}
};
import io.hansel.ujmtracker.HanselInternalEventsListener
var hanselInternalEventsListener : HanselInternalEventsListener = object : HanselInternalEventsListener() {
fun onEvent(eventName:String, dataFromHansel:HashMap<String, Any>) {
//Fire this hansel event using your analytics vendor as explained in the above section (Handling existing app events)
//e.g. AppCleverTap.push(clevertapAPI, eventName, properties)
//In case your app is using multiple analytics vendors, then please fire the hansel events with only one vendor
}
}
Step 2 : Registering the listener with Nudge SDK
import io.hansel.ujmtracker.HanselTracker;
//Register the listener with Hansel SDK by using this code.
HanselTracker.registerListener(hanselInternalEventsListener);
import io.hansel.ujmtracker.HanselTracker
//Register the listener with Hansel SDK by using this code.
HanselTracker.registerListener(hanselInternalEventsListener)
Note
- Please ensure to select the checkbox 'Send nudges to Analytics Provider' from Step 2 of Nudge creation flow.
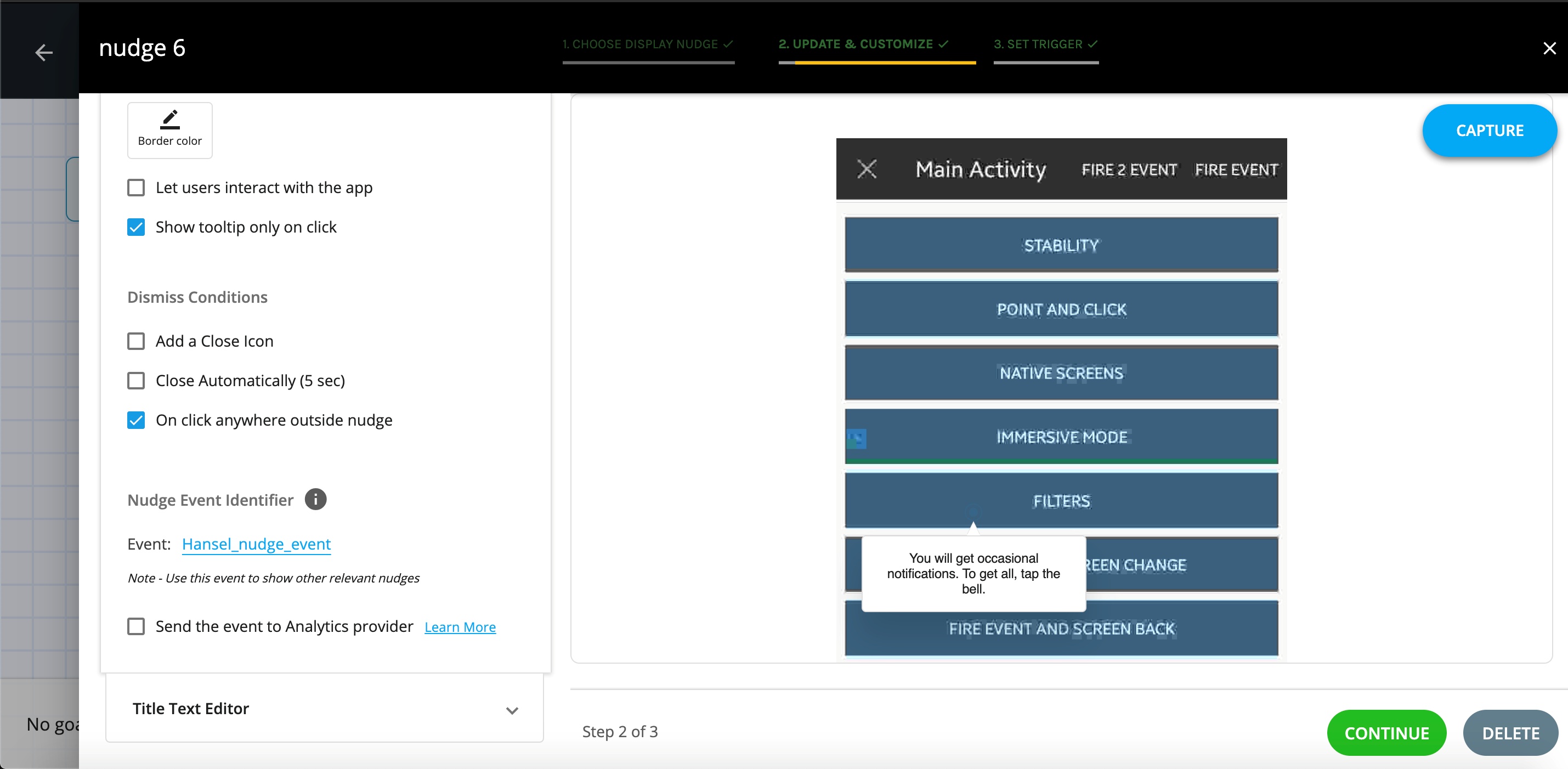
- Below are properties that you can expect in 'hansel_nudge_event'
- interaction_map_name
- nudge_name
- nudge_type
- app_id
- user_id (fallback to device id if not present)
- nudge_rating : NULL | Rating | NPS (will be a number)
- nudge_mcq : MCQ (will be a string)
- nudge_inputtext: NULL | text
- nudge_action: button1/2_clicked | buttontext | close_clicked | noaction_autodismissed | noaction_screenchanged | noaction_appclosed | backdrop_dismissed
Possible values for nudge_action:
- auto dismissed (5 sec) - noaction_autodismissed
- app crash/ app killed -noaction_appclosed
- out of view port/SDK methods (in case of web), orientation change (landscape to portrait) -noaction_screenchanged
- button_action: NULL / Close / Action / URL
- time_spent_nudge - in seconds (will be a number)
Updated over 4 years ago